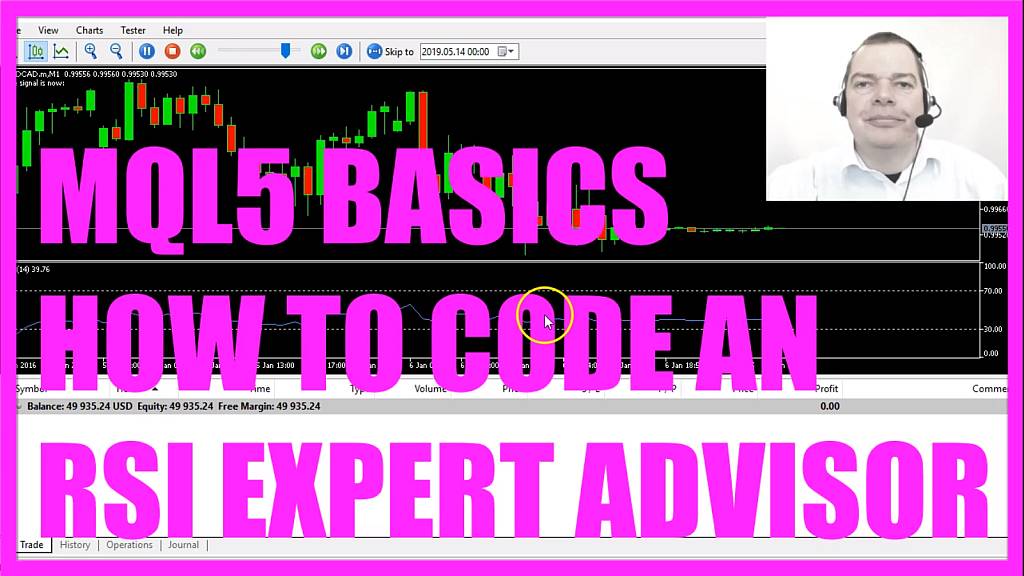
- Introduction to Creating an RSI Trading Expert Advisor in MQL5 (00:01 – 00:16)
- Introduction to the tutorial on creating an Expert Advisor for trading the Relative Strength Index (RSI) using MQL5.
- Opening MetaEditor and Creating a New Expert Advisor File (00:16 – 00:38)
- Instructions on opening MetaEditor and creating a new Expert Advisor file named “SimpleRSIEA”.
- Setting Up the Code Structure and Including Trade.mqh (00:38 – 01:01)
- Removing unnecessary code and including the ‘Trade.mqh’ file for trading functions.
- Calculating Ask and Bid Prices (01:01 – 01:29)
- Using ‘SymbolInfoDouble’ to calculate the Ask and Bid prices and normalizing them for currency pair precision.
- Creating a Variable for the Signal and Price Array (01:29 – 02:06)
- Initializing a variable for the trading signal and creating an array for price data called “myRSIArray”.
- Defining RSI Properties and Filling the Price Array (02:06 – 03:27)
- Defining properties for the RSI and using ‘CopyBuffer’ to fill the price array with RSI data.
- Determining Buy and Sell Signals Based on RSI (03:27 – 04:39)
- Setting conditions for buy and sell signals based on the RSI value relative to overbought and oversold levels.
- Executing Trades Based on Signals (04:39 – 05:06)
- Using ‘trade.Sell’ and ‘trade.Buy’ to execute trades based on the determined signals.
- Displaying the Current Signal on the Chart (05:06 – 05:18)
- Using the ‘Comment’ function to display the current trading signal on the chart.
- Compiling the Code and Testing in MetaTrader (05:18 – 05:45)
- Compiling the code and testing the Expert Advisor in MetaTrader using the Strategy Tester.
- Observing the RSI EA in Action on the Chart (05:45 – 07:00)
- Watching the Expert Advisor trade the RSI on the chart and analyzing its performance.
This overview provides a detailed summary of the video’s content, organized into specific chapters with corresponding timestamps.
In this video, we are going to create an Expert Advisor to automatically calculate and trade the Relative Strength Index also called RSI, so let’s find out how to do that with MQL5.
First you need to click on the little button here or press F4 on your keyboard, now you should see the Metaeditor window and here you want to click on: “File/ New File/ Expert Advisor (template)” from template, “Continue”, I will call this file: “SimpleRSIEA”, click on “Continue”, “Continue” and “Finish”.
Now you can delete everything above the “OnTick” function and the two comment lines here.
We start with an include statement, we want to include the file: “Trade.mqh”, it comes with trading functions and it is included in MQL5, so now we can create an instance called: “trade”.
Inside of the “OnTick” function we want to calculate the Ask price and the Bid price because we are going to buy and sell, so we use “SymbolInfoDouble”, “_Symbol“, “SYMBOL_ASK” to calculate the Ask price and with “SymbolInfoDouble”, “_Symbol“, “SYMBOL_BID” – all in capital letters – we will get the Bid price.
“NormalizeDouble” and “_Digits” is a way to automatically calculate the number of digits behind the dot because the “_Digits” variable stores the number of digits after the decimal point that might be 3 digits – like in this case – or 5 digits.
Let’s create a variable for the signal, the name is also “signal”, please don’t assign a value right now because we need to calculate that.
We need to create an array for the price data that will be called: “myRSIArray”, it’s a double array, so let’s define the properties of the RSI (myRSIDefinition), we have an included function in MQL5 that is called: “iRSI”, it takes a few parameters;
The first parameter is for the current symbol on the chart,
The second one for the period,
This value; 14, is also what you see if you click on: “Insert/ Indicators/ Oscillators/ Relative Strength Index”, here you have a period value for 14 candles and it is applied to the close prices.
This is how the Indicator looks like.
Until the last major update, it was automatically shown on the chart whenever I calculated it, but now I need to right-click into the chart, select “Templates/ Save Template” and save it as: “tester.tpl” because this is the template that is going to be used in the backtest and the default template is the one that you will see when you drag any Expert Advisor on the chart, so let’s choose “tester.tpl” and save it.
Yes, I want to override it, so this parameter is for the period,
This one is to calculate values based on the close price.
Now I use “CopyBuffer” to fill the price array (myRSIArray) that we have created here with data according to the definition (myRSIDefinition) that we made here.
This parameter here stands for the buffer. The buffer is in our case the blue line, this parameter here stands for the current candle 0 (zero), we want to calculate the values for 3 candles and store them in the RSI array (myRSIArray) and that will make it possible to calculate an RSI value (myRSIValue) for the current candle by simply looking into candle 0 (zero) of our RSI array (myRSIArray), “NormalizeDouble” and comma 2 is used to cut the output to two digits like in the Indicator and if the RSI value(myRSIValue) is bigger than 70 – that’s the case when it crosses this line – and is above we consider the value to be overbought, so we want to assign “sell” to our signal.
In the other case if the RSI value(myRSIValue) is below 30 we want to buy because when it’s below this line we think that it is oversold.
So if the signal equals “sell” and if “PositionsTotal” is below 1 – so we have no open positions – we want to use “trade.Sell” to sell 10 micro lot.
Otherwise, if the signal equals “buy” and we have no open positions we use “trade.Buy” and buy 10 micro lot.
Finally, we use the “Comment” statement to output the text: “The signal is now:” followed by the current signal.
Okay, that’s about it.
If this was too fast for you or if you don’t know what the code does, I would suggest watching the other basic tutorial videos or maybe you are even interested in the premium course, for now, click on the “Compile” button, you shouldn’t get any errors here and if that is the case you can click the little button here or press F4 to go back to Met trader.
Please don’t forget to save the current chart as “tester.tpl”, click on: “View/ Strategy Tester” or press CTRL and R, now you should see the Strategy Tester and here you want to pick the file: “SimpleRSIEA.ex5”, please mark the option for the visualization here and start a test.
…and here is our little Expert Advisor running, I now see two RSI Oscillators here…
I… think I know that I forgot to use the “ArraySetAsSeries” statement to solve the current array, so let’s do that in the source code.
“ArraySetAsSeries” is used to sort the array from the current candle downwards and that actually seems to affect the Strategy Tester template, so let’s recompile the code here, stop the current test, click on the “Start” button and this time our Expert Advisor is working as expected, it generates buy and sell signals and it is also able to automatically trade them, and you have learned how to do that yourself and you coded it with a few lines of MQL5 code.