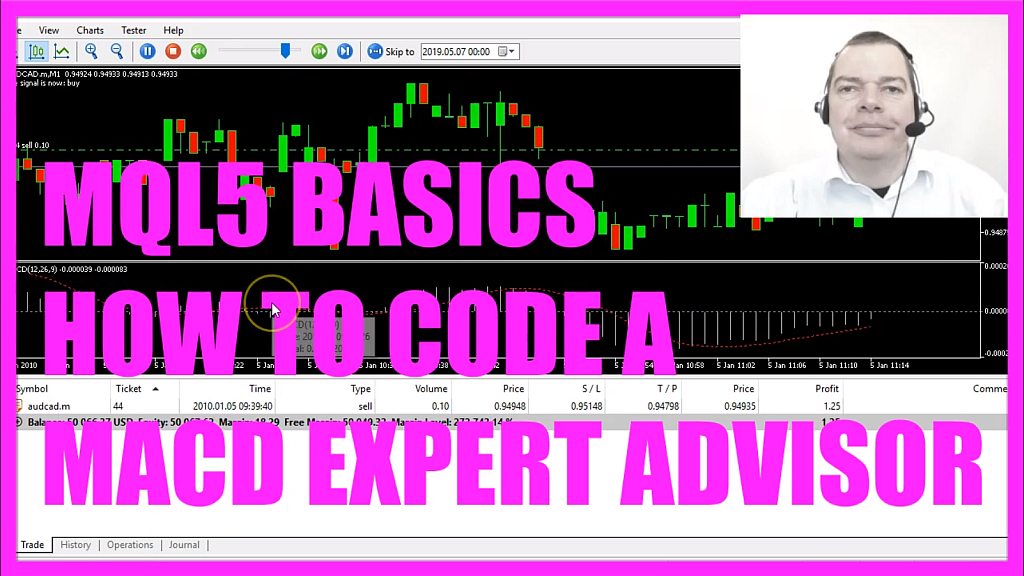
- Introduction to Creating a MACD Trading Expert Advisor in MQL5 (00:01 – 00:08)
- Introduction to the tutorial on automating MACD trading using MQL5.
- Opening MetaEditor and Creating a New Expert Advisor File (00:08 – 00:34)
- Instructions on opening MetaEditor and creating a new Expert Advisor file named “SimpleMacDEA”.
- Setting Up the Code Structure and Including Trade.mqh (00:34 – 00:58)
- Removing unnecessary code and including the ‘Trade.mqh’ file for trading functions.
- Calculating Ask and Bid Prices (00:58 – 01:24)
- Using ‘SymbolInfoDouble’ to calculate the Ask and Bid prices and normalizing them for currency pair precision.
- Creating a String for the Signal and Price Array (01:24 – 01:44)
- Initializing a string for the trading signal and creating an array for price data.
- Defining MACD Properties and Filling the Price Array (01:44 – 03:08)
- Defining properties for the MACD and using ‘CopyBuffer’ to fill the price array with MACD data.
- Determining Buy and Sell Signals Based on MACD (03:08 – 04:09)
- Setting conditions for buy and sell signals based on the MACD value relative to the zero line.
- Executing Trades Based on Signals (04:09 – 04:38)
- Using ‘trade.Sell’ and ‘trade.Buy’ to execute trades based on the determined signals.
- Creating Chart Output for the Signal (04:38 – 04:48)
- Using the ‘Comment’ function to display the current trading signal on the chart.
- Compiling the Code and Testing in MetaTrader (04:48 – 05:25)
- Compiling the code and testing the Expert Advisor in MetaTrader using the Strategy Tester.
- Observing the MACD EA in Action on the Chart (05:25 – 05:50)
- Watching the Expert Advisor trade the MACD on the chart and analyzing its performance.
In this video, we are going to create an Expert Advisor that is actually going to trade the MACD.
The MACD is an oscillator and now we want to automate it with MQL5.
To do that please click on the little button here or press F4 in your Metatrader, now you should see the Metaeditor window and here you want to click on: “File/ New File/ Expert Advisor (template)” from template, “Continue”, I will call this file: “SimpleMacDEA”, click on “Continue”, “Continue” and “Finish”.
Now you can remove everything above the “OnTick” function and the two comment lines here.
We start by including the file: “Trade.mqh”, this one will give us some trading functions, afterwards we will create an instance of “CTrade” that will be called: “trade” and we are going to use it to open positions later on.
To be able to do that we need to calculate the Ask price and the Bid price that is done by using “SymbolInfoDouble” for the current symbol on the chart, we use “SYMBOL_ASK” or “SYMBOL_BID” and with “NormalizeDouble” and “_Digits” we make sure that we are getting either 3 or 5 digits behind the dot depending on the currency pair we used that might be different.
I would like to create a string for the signal that will be called: “signal”, it doesn’t have any value right now, we need to calculate that value later on.
Let’s create an array for several prices that will be called: “myPriceArray“, now we defined the properties for the MACD Expert Advisor (MacDDefinition), MQL5 comes with an included function called: “iMACD”, it takes a few parameters:
The first one is for the current symbol on the chart,
The second one is for the period that is selected on that chart,
Here we have 3 values and the MACD value should be calculated based on the close price, so let’s find out what these 3 values mean.
On any chart click on: “Insert/ Indicators/ Oscillators/ MACD” and you will see the 3 values: 12, 26 and 9. 12 is the value for the fast Exponential Moving Average (Fast EMA), 26 is the value for the slow Exponential Moving Average (SlowEMA) and there is also a value of 9 for the MACD Simple Moving Average (MACD SMA), if you are interested in the exact formula you could go to sites like investopedia.com. What’s important for now is that the 3 values are used here to calculate one value, we use “CopyBuffer” to copy the price data into our price array (myPriceArray), we do it according to the MACD definition (MacDDefinition) that we have created here for the first buffer – let’s actually call butter – we start with the current candle 0 (zero) and we copy the values for 3 candles.
From time to time people ask me why I always use 3 candles, well that’s handy if you want to calculate a crossover value and it’s much faster than it would be to use all the candles on the chart, so we can save some processing power here.
Now I can get the value for the MACD by looking into the value of candle 0 (zero) of our price array (myPriceArray), for this simple example let’s assume that we have a sell signal whenever the MACD value (MacDValue) is above the 0 (zero) line – this is the zero line – and when the MACD value (MacDValue) is below the zero line that would be a buy signal and when our signal equals sell and “PositionsTotal” is below 1 – that would mean we have no open position – we would use “trade.Sell” to sell 10 micro lot.
In the other case if the signal equals buy and we have no open positions we would use “trade.Buy” and buy 10 micro lot.
Finally, we want to create a chart output, I would like to see the text: “The signal is now:” followed by the signal that we have created. That’s about it, so let’s click on the “Compile” button here, I have one warning because I use a float variable here, this one will give me fewer digits behind the dot but it’s faster and it’s enough for our simple example here, so if you don’t have any errors you can click on the little button here or press F4 to go back to Metatrader.
And in Metatrader we click on: “View/ Strategy Tester” or press CTRL and R, here you want to pick the file: “SimpleMacDEA.ex5”, please mark the visualization option here and start a test.
Now you should actually see the MACD and here is our first position, it’s actually trading and in this little video you have learned how to automate the MACD and create an MQL5 Expert Advisor that is actually going to trade it and you have coded it yourself with a few lines of MQL5 code.
Download “CODE - SIMPLE MACD EA”
SimpleMacDEA.mq5 – Downloaded 87 times – 1.43 KB